A few weeks ago, I have shown you the steps of creating a Singleton object for Flex applications. As for this week, I am going to show you how to create a Singleton for a Android Application.
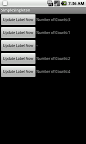
Image of Demo Android App.
The App that I am showing in this post will be using the Singleton
object to store the number of clicks.
Main application file - zcs/simpleSingleton/SimpleSingletonActivity.java
package zcs.simpleSingleton;
import android.app.Activity;
import android.os.Bundle;
public class SimpleSingletonActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
A reusable Custom Component File - zcs/simpleSingleton/CustomComponent.java
package zcs.simpleSingleton;
import zcs.singleton.MainSingleton;
import android.content.Context;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.TextView;
public class CustomComponent extends LinearLayout
{
private TextView txt = null;
public CustomComponent(Context context, AttributeSet attrs) {
super(context, attrs);
// TODO Auto-generated constructor stub
LayoutInflater.from(context).
inflate(R.layout.layout_custom_component, this, true);
Button btn = (Button) this.findViewById(R.id.btn);
btn.setOnClickListener(clickListener);
txt = (TextView) this.findViewById(R.id.txt);
}
// Create a clickListener of type OnClickListener
// Which will be added to the Button
private OnClickListener clickListener = new OnClickListener()
{
@Override
public void onClick(View arg0) {
//Create an instance of MainSingleton
MainSingleton tempObj;
tempObj = MainSingleton.getInstance();
//Get the current value for Counter and
//increase it by 1
int tempCount = tempObj.getCounter() + 1;
//Set it back to the MainSingleton
tempObj.setCounter(tempCount);
//Update the text of the label
txt.setText("Number of Counts:" + tempCount);
}
};
}
Main Singleton File - zcs/singleton/MainSingleton.java
package zcs.singleton;
public class MainSingleton
{
private static MainSingleton instance;
public static MainSingleton getInstance()
{
//If the Singleton is not found, create
//an instance of it and init / reset the
//values.
if(instance == null)
{
instance = new MainSingleton();
instance.reset();
}
return instance;
}
//function to reset data
public void reset()
{
_counter = 0;
}
//Variable storing the number of counter
private int _counter = 0;
public int getCounter() {
return _counter;
}
public void setCounter(int _counter) {
this._counter = _counter;
}
}
Main Application XML Layout file - layout.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<zcs.simpleSingleton.CustomComponent
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TableLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<zcs.simpleSingleton.CustomComponent
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<zcs.simpleSingleton.CustomComponent
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
<TableLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<zcs.simpleSingleton.CustomComponent
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<zcs.simpleSingleton.CustomComponent
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
</TableLayout>
</TableLayout>
</LinearLayout>
Custom Component Layout File
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<Button android:id="@+id/btn"
android:text="@string/btn_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView android:id="@+id/txt"
android:text="@string/txt_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
* Click
here to download the source files of this Android Demo.